SightMap® JavaScript IFrame API
Introduction
The SightMap® JavaScript IFrame API provides third-party developers the ability to deeply integrate SightMap®. Deeper integration can enable rich user experiences and allow websites or applications to move beyond typical integration standards.
Quick Start
A working example integrating the API with an example SightMap instance is below. You may insert the following usage examples within this HTML document in order to explore the API.
Setting the origin valueSet the
origin
query parameter to the origin serving the document. The example useshttp://localhost:3000
, however please update the value accordingly.If you need to start a local server, the following gist provides instructions for doing so quickly on either Mac or PC: https://gist.github.com/jgravois/5e73b56fa7756fd00b89.
For security reasons, the example must be served from an origin and will not function correctly when directly opened in a Browser.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>SightMap JavaScript IFrame API Example</title>
<style type="text/css">
* {
box-sizing: border-box;
}
html, body {
height: 100%;
margin: 0;
padding: 0;
}
</style>
</head>
<body>
<!-- The API script must be included on the page -->
<script src="https://sightmap.com/embed/api.js"></script>
<!--
Include the IFrame embed code. The embed URL must include `enable_api=1` and
provide the pages origin, the example uses `http://localhost:3000`, however
update this accordingly. Additionally, the iframe must declare an id
attribute, in this case it is set to `sightmap`.
-->
<iframe id="sightmap" src="https://sightmap.com/embed/ryzvg6mywln?enable_api=1&origin=http://localhost:3000" frameborder="0" width="100%" height="100%"></iframe>
<script type="text/javascript">
// Create a new embed instance, providing the IFrame id value:
var embed = new SightMap.Embed('sightmap');
embed.on('ready', function () {
// Usage example code goes here...
});
</script>
</body>
</html>
Usage Requirements
In order to use the SightMap JavaScript IFrame API, the page must include the following script tag prior to the embed code.
<!-- The API script must be included on the page -->
<script src="https://sightmap.com/embed/api.js"></script>
The embed url must also pass parameters to enable the API and specify the web page origin. An id must also be declared, to be referenced when initializing the Embed object.
<iframe id="sightmap" src="https://sightmap.com/embed/dgow3krqp2m?enable_api=1&origin=http://localhost:3000" frameborder="0" width="100%" height="100%"></iframe>
setFloor
Set the active Floor by given ID.
TypeScript Definition
setFloor(id: string): void
// Set the active floor to the 2nd floor, which has an ID of `4731`:
embed.setFloor('4731');
getFloor
Retrieve the active floor ID if set.
TypeScript Definition
getFloor(callback: (id?: string) => void): void
// Get the active floor ID:
embed.getFloor(function (id) {
console.log('Active Floor ID:', id);
});
locateUnitById
Locate and activate a Unit by ID.
TypeScript Definition
locateUnitById(id: string, options?: object): void
// Example 1: Locate unit E05, which has ID `258702`.
embed.locateUnitById('258702');
// Example 2: Locate unit `258702` and display the Unit Details modal.
embed.locateUnitById('258702', {displayDetails: true});
Options
Option | Type | Default Value | Description |
---|---|---|---|
displayDetails | Boolean | false | Determines if the Unit Details modal is displayed. |
locateUnitByUnitNumber
Locate and activate a Unit by unit number.
TypeScript Definition
locateUnitByUnitNumber(unitNumber: string, options?: object): void
Unit Number UniquenessThere are SightMap instances in which unit numbers are not unique within the set of units. Use the
locateUnitById
method in these cases.To obtain unit ID values, please contact your SightMap Integration Specialist.
// Example 1: Locate unit `E05`.
embed.locateUnitByUnitNumber('E05');
// Example 2: Locate unit `E05` and display the Unit Details modal.
embed.locateUnitByUnitNumber('E05', {displayDetails: true});
Options
Option | Type | Default Value | Description |
---|---|---|---|
displayDetails | Boolean | false | Determines if the Unit Details modal is displayed. |
setUnitIdMatches
Provide a custom result set of unit ID values to filter on, known as "Your Matches".
TypeScript Definition
setUnitIdMatches(unitIds: string[], options?: object): void
// Example 1: Set matches to the given units based on id.
var ids = [
'258780', // W04
'258702', // E05
'258748', // E10
'258672', // SE100
'258759', // W22
];
embed.setUnitIdMatches(ids);
// Example 2: Set matches to the given units based on id and display filter input.
var ids = [
'258780', // W04
'258702', // E05
'258748', // E10
'258672', // SE100
'258759', // W22
];
embed.setUnitIdMatches(ids, {filterEditing: true});
// Example 3: Display 0 matches by providing an empty array of id values.
var ids = [];
embed.setUnitIdMatches(ids);
Options
Option | Type | Default Value | Description |
---|---|---|---|
filterEditing | Boolean | false | Determines if filter input is displayed. |
setUnitNumberMatches
Provide a custom result set of unit number values to filter on, known as "Your Matches".
TypeScript Definition
setUnitNumberMatches(unitNumbers: string[], options?: object): void
Unit Number UniquenessThere are SightMap instances were unit numbers are not unique within the set of units. Use the
setUnitIdMatches
method in these cases.To obtain unit ID values, please contact your SightMap Integration Specialist.
// Example 1: Set matches to the given units based on unit number.
var unitNumbers = [
'W04',
'E05',
'E10',
'SE100',
'W22'
];
embed.setUnitNumberMatches(unitNumbers);
// Example 2: Set matches to the given units based on unit number and display filter input.
var unitNumbers = [
'W04',
'E05',
'E10',
'SE100',
'W22'
];
embed.setUnitNumberMatches(unitNumbers, {filterEditing: true});
// Example 3: Display 0 matches by providing an empty array of unit number values.
var unitNumbers = [];
embed.setUnitNumberMatches(unitNumbers);
Options
Option | Type | Default Value | Description |
---|---|---|---|
filterEditing | Boolean | false | Determines if filter input is displayed. |
getUnitIds
Retrieve a list of unit IDs from the set of units.
TypeScript Definition
getUnitIds(callback: (unitIds: string[]) => void): void
// Get the list of unit IDs:
embed.getUnitIds(function (unitIds) {
console.log('Unit IDs:', unitIds);
});
getUnitNumbers
Retrieve a list of unit numbers from the set of units.
TypeScript Definition
getUnitNumbers(callback: (unitNumbers: string[]) => void): void
// Get the list of unit numbers:
embed.getUnitNumbers(function (unitNumbers) {
console.log('Unit Numbers:', unitNumbers);
});
Unit Number UniquenessThere are SightMap instances in which unit numbers are not unique within the set of units. Therefore, this method may return duplicate values.
If unique values are required or preferred, use the
getUnitIds
method instead. To obtain unit ID values, please contact your SightMap Integration Specialist.
getMapView
Retrieve the active map view selected.
TypeScript Definition
getMapView(callback: (mapView: { type: string }) => void): void
// Get the active map view selection:
embed.getMapView(function (mapView) {
console.log('Active map view:', mapView.type);
});
mapView
Object Key | Type | Description |
---|---|---|
type | string | Determines the active map view selected. |
disableUI
Disable certain UI elements.
TypeScript Definition
disableUI(elements: string[]): void
/* Disable certain UI elements
'filters' hides the filter section
'unitList' hides the unit list section
'floorSelection' hides the floor selector
'unitTooltip' disables the unit hover tooltip.
'unitDetails' disables the unit details modal.
*/
embed.disableUI(['filters','unitList','floorSelection','unitToolTip','unitDetails']);
Floor NavigationDo not hide the floor selector unless you have created a alternate way to identify the floors. Exceptions may be made for properties that have only ONE floor, and there are NO other floors in the building(s).
Events
ready
ready
The ready
event fires when the embed has loaded enough to allow for API input and/or user interaction.
embed.on('ready', function () {
console.log('The embed is ready.')
});
outbound.{key}.click
outbound.{key}.click
The outbound.{key}.click
event fires when a call-to-action (CTA) configured for the IFrame API is selected by the user.
{key}
would be replaced by the specific key Engrain configures on your CTA. For instance, partnerAction
is the key in the usage example below.
This is only available if an IFrame API CTA has been configured for you by the Engrain team. Please reach out to the Engrain team if you are interested in a CTA API interaction.
{
name: "outboundLink.partnerAction.click",
data: {
unit: {
id: "374030",
mapId: "374030",
unitNumber: "2-101",
unitNumberDisplay: "APT 1031"
area: 1887,
areaDisplay: "1,877 Sq. Ft.",
price: 2315, // nullable
priceDisplay: "$2,315", // nullable
availableOn: "2018-09-14", // nullable
availableOnDisplay: "Available Now", // nullable
floor: {
id: "7961",
filterLabel: "Floor 1",
filterShortLabel: "1"
},
floorPlan: {
id: "25575",
name: "A2",
bedroomCount: 1,
bathroomCount: 1
}
}
}
}
embed.on('outbound.partnerAction.click', function(event) {
// Your desired result goes here
});
unitDetails.show
unitDetails.show
The unitDetails.show
event fires when a unit is clicked from the Unit List or the Map section of the embed.
Heads upThis event will only fire if the unitDetails modal is disabled. You can disable it with:
embed.disableUI(['unitDetails'])
.
{
name: "uniDetails.show",
data: {
unit: {
id: "374030",
unitNumber: "2-101",
unitNumberDisplay: "APT 1031"
area: 1887,
areaDisplay: "1,877 Sq. Ft.",
price: 2315, // nullable
priceDisplay: "$2,315", // nullable
availableOn: "2018-09-14", // nullable
availableOnDisplay: "Available Now", // nullable
floor: {
id: "7961",
filterLabel: "Floor 1",
filterShortLabel: "1"
},
floorPlan: {
id: "25575",
name: "A2",
bedroomCount: 1,
bathroomCount: 1
}
}
}
}
embed.on('unitDetails.show', function(event) {
// Your code here
});
mapUnit.mouseEnter
mapUnit.mouseEnter
The mapUnit.mouseEnter
event fires when a unit is hovered on the Map section of the embed.
Heads upThis event will only fire if the unitToolTip is disabled. You can disable it with:
embed.disableUI(['unitToolTip'])
.This event should be used in conjunction with the
'mapUnit.mapLeave'
event.
{
name: "mapUnit.mouseEnter",
data: {
unit: {
id: "374030",
unitNumber: "2-101",
unitNumberDisplay: "APT 1031"
area: 1887,
areaDisplay: "1,877 Sq. Ft.",
price: 2315, // nullable
priceDisplay: "$2,315", // nullable
availableOn: "2018-09-14", // nullable
availableOnDisplay: "Available Now", // nullable
floor: {
id: "7961",
filterLabel: "Floor 1",
filterShortLabel: "1"
},
floorPlan: {
id: "25575",
name: "A2",
bedroomCount: 1,
bathroomCount: 1
}
}
mouseX: 100, // Pixels from the left edge of the iframe window to the mouse's X coordinate
mouseY: 200, // Pixels from the top edge of the iframe window to the mouse's Y coordinate
}
}
embed.on("mapUnit.mouseEnter", (event) => {
// Your code here
});
mapUnit.mouseLeave
mapUnit.mouseLeave
The mapUnit.mouseLeave
event fires when a hovered unit is exited on the Map section of the embed.
Heads upThis event will only fire if the unitToolTip is disabled. You can disable it with:
embed.disableUI(['unitToolTip'])
.This event should be used in conjunction with the
'mapUnit.mouseEnter'
event.
{
name: "mapUnit.mouseLeave",
data: {
unit: {
id: "374030",
unitNumber: "2-101",
unitNumberDisplay: "APT 1031"
area: 1887,
areaDisplay: "1,877 Sq. Ft.",
price: 2315, // nullable
priceDisplay: "$2,315", // nullable
availableOn: "2018-09-14", // nullable
availableOnDisplay: "Available Now", // nullable
floor: {
id: "7961",
filterLabel: "Floor 1",
filterShortLabel: "1"
},
floorPlan: {
id: "25575",
name: "A2",
bedroomCount: 1,
bathroomCount: 1
}
}
mouseX: 100, // Pixels from the left edge of the iframe window to the mouse's X coordinate
mouseY: 200, // Pixels from the top edge of the iframe window to the mouse's Y coordinate
}
}
embed.on("mapUnit.mouseLeave", (event) => {
// Your code here
});
mapUnit.mouseMove
mapUnit.mouseMove
The mapUnit.mouseMove
event fires any time the cursor is moved within the boundaries of a unit in the embed.
Heads upThis event will only fire if the unitToolTip is disabled. You can disable it with:
embed.disableUI(['unitToolTip'])
.This event should be used in conjunction with the
'mapUnit.mouseEnter'
and the'mapUnit.mouseLeave'
events.
{
name: "mapUnit.mouseMove",
data: {
unit: {
id: "374030",
unitNumber: "2-101",
unitNumberDisplay: "APT 1031"
area: 1887,
areaDisplay: "1,877 Sq. Ft.",
price: 2315, // nullable
priceDisplay: "$2,315", // nullable
availableOn: "2018-09-14", // nullable
availableOnDisplay: "Available Now", // nullable
floor: {
id: "7961",
filterLabel: "Floor 1",
filterShortLabel: "1"
},
floorPlan: {
id: "25575",
name: "A2",
bedroomCount: 1,
bathroomCount: 1
}
}
mouseX: 100, // Pixels from the left edge of the iframe window to the mouse's X coordinate
mouseY: 200, // Pixels from the top edge of the iframe window to the mouse's Y coordinate
}
}
embed.on("mapUnit.mouseMove", (event) => {
// Your code here
});
floor.change
floor.change
The floor.change
event fires when the floor has changed in the embed.
embed.on('floor.change', function(event) {
console.log('floor id is now: ', event.id)
})
mapView.change
mapView.change
The mapView.change
event fires when the map view has changed in the embed.
embed.on('mapView.change', function(event) {
console.log('map view is now: ', event)
})
Examples
disableUI
This is an example of a SightMap using the disableUI
call.
Heads up: Maps in the mirror are larger than they appear.The size of the map in these JSFiddles is scaled to the size of the example window. As such, the size of the map will be much larger on an actual website.
Combined Demo
This examples gives one the ability to set unit matches, locate units, switch floors, and disable certain UI elements. This example uses jQuery.
Heads up: Maps in the mirror are larger than they appear.The size of the map in these JSFiddles is scaled to the size of the example window. As such, the size of the map will be much larger on an actual website.
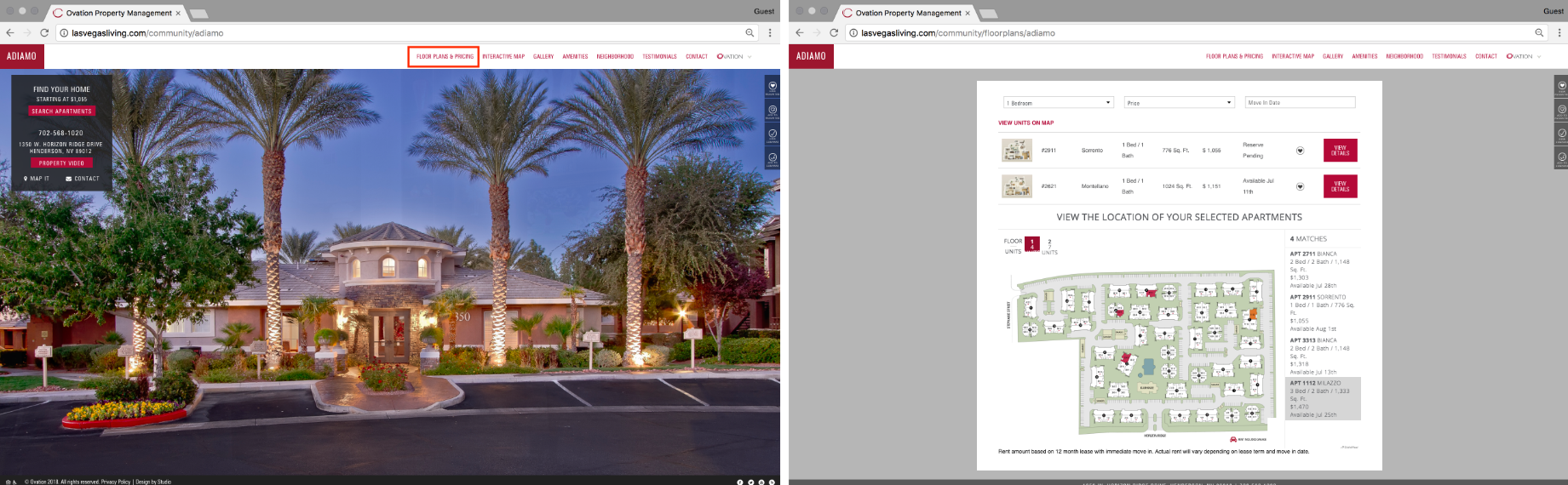
Updated 4 months ago